1
Please generate a random float where the value is between 5 and 95 using Python math module.
Use random.random() to generate a random float in [0,1].
分析:random.random()可以生成随机数
1 | import random |
2
Please generate a random float where the value is between 10 and 100 using Python math module.
1 | import random |
3
Please write a binary search function which searches an item in a sorted list. The function should return the index of element to be searched in the list.
分析:二分查找
1 | li =[1,3,4,6,8,10,15] |
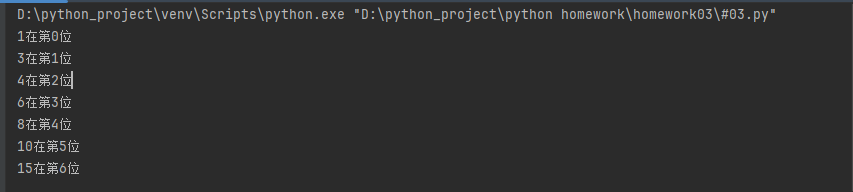
4
Please write a binary search function which searches an item in a sorted list. The function should return the index of element to be searched in the list.
分析:二分查找
1 | li =[1,3,4,6,8,10,15] |
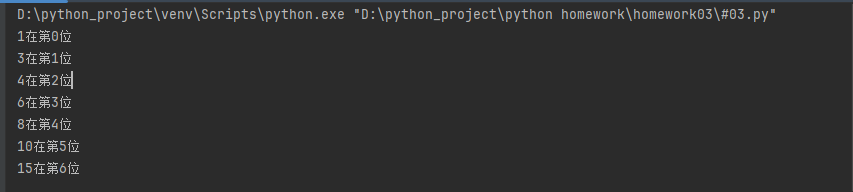
5
Please write a program which accepts basic mathematic expression from console and print the evaluation result.
分析:eval()可以把读入的字符串转换为数学表达式并计算
1 | print(eval(input("请输入数学表达式:"))) |
6
Please write assert statements to verify that every number in the list [2,4,6,8] is even.
分析:Python assert(断言)用于判断一个表达式,在表达式条件为 false 的时候触发异常。
1 | li=[2,4,6,8] |
7
Please write a program using generator to print the numbers which can be divisible by 5 and 7 between 0 and n in comma separated form while n is input by console.
分析:yield 的作用就是把一个函数变成一个 generator,带有 yield 的函数不再是一个普通函数,Python 解释器会将其视为一个 generator
1 | n = input("Please input n:") |
8
Please write a program using generator to print the even numbers between 0 and n in comma separated form while n is input by console.
1 | n = input("please input n:") |
9
The Fibonacci Sequence is computed based on the following formula:
f(n)=0 if n=0
f(n)=1 if n=1
f(n)=f(n-1)+f(n-2) if n>1
Please write a program using list comprehension to print the Fibonacci Sequence in comma separated form with a given n input by console.
分析:递归
1 | li=[] |
10
The Fibonacci Sequence is computed based on the following formula:
f(n)=0 if n=0
f(n)=1 if n=1
f(n)=f(n-1)+f(n-2) if n>1
Please write a program to compute the value of f(n) with a given n input by console.
1 | def fun( n ): |
11
Write a program to compute:
f(n)=f(n-1)+100 when n>0 and f(0)=1
with a given n input by console (n>0).
1 | def f(n): |
12
Write a program to compute 1/2+2/3+3/4+…+n/n+1 with a given n input by console (n>0).
分析:递归
1 | def f(n): |
13
Write a program to read an ASCII string and to convert it to a unicode string encoded by utf-8.
1 | s = input("input:") |
14
Write a program which accepts a sequence of words separated by whitespace as input to print the words composed of digits only.
分析:考察正则表达式 re.findall()可以查找字符串中所有匹配模式的子串,并以列表的形式存储
1 | import re |
15
Assuming that we have some email addresses in the “username@companyname.com“ format, please write program to print the company name of a given email address. Both user names and company names are composed of letters only.
分析:正则表达式,re.sub()可以替换掉匹配模式的字符串
1 | import re |
16
Assuming that we have some email addresses in the “username@companyname.com“ format, please write program to print the user name of a given email address. Both user names and company names are composed of letters only
1 | import re |
17
Define a custom exception class which takes a string message as attribute.
1 | class MyError(Exception): |
18
Write a function to compute 5/0 and use try/except to catch the exceptions.
分析:考察 异常
1 | def f(): |
19
Define a class named Shape and its subclass Square. The Square class has an init function which takes a length as argument. Both classes have a area function which can print the area of the shape where Shape’s area is 0 by default.
分析:考察类的继承 和 方法的覆写
1 | class Shape (object): |
20
Define a class named Rectangle which can be constructed by a length and width. The Rectangle class has a method which can compute the area.
1 | class Rectangle(object): |
转载请注明来源,欢迎对文章中的引用来源进行考证,欢迎指出任何有错误或不够清晰的表达。可以在下面评论区评论