java注解与反射
发布时间 :
字数:3.5k
阅读 :
文章来源:Java注解与反射——看完这一篇就够了 - Devin-Y - 博客园 (cnblogs.com)
视频:B站_注解与反射
注解(Annotation)与反射(Reflection)
一、注解
1.1、什么是注解
- Annotation是JDK.5.0开始引入的技术
- Annotation的作用:
- 不是程序本身,可以对程序做出解释。(这一点和注释(comment)没有什么区别)
- 可以被其他程序(比如:编译器)读取
- Annotation的格式:
- 注解是以”@注释名”在代码中存在的,还可以添加一些参数值,例如:@SuppressWarnings(value=”unchecked”);
- Annotation在哪里使用?
- 可以附加在package,class,method,field等上面,相当于给它们添加了额外的辅助信息,我们可以通过反射机制编程实现对这些元数据的访问
1 2 3 4 5 6 7 8
| public class Test01 extends Object{ @Override public String toString() { return super.toString(); } }
|
1.2、内置注解
@Override:定义在java.lang.Override中,此注释只适用于修饰方法,表示一个方法声明打算重写超类中的另一个方法声明;
@Deprecated:定义在java.lang.Deprecated中,此注释可以用于修饰方法、属性、类,表示不鼓励程序员使用这样的元素,通常是因为它很危险或者存在更好的选择。
@SuppressWarnings:定义在java.lang.SuppressWarnings中,用来抑制编译时的警告信息。
- 与上面两个注释有所不同,你需要添加一个参数才能正确使用,这些参数都是已经定义好了的,我们选择使用就行!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| @SuppressWarnings("all") @SuppressWarnings("unchecked") @SuppressWarnings(value={"unchecked","Deprecated"}) ......
public class Test01 extends Object{ @Override public String toString() { return super.toString(); }
@Deprecated public static void test(){ System.out.println("test-------->Deprecated"); }
@SuppressWarnings("all") public void test02(){ List list = new ArrayList(); }
public static void main(String[] args) { test(); } }
|
1.3、元注解
- 元注解的作用就是负责注解其他注解,Java定义了4个标准的meta-annotation类型,他们被用来提供对其他annotation类型作说明
- 这些类型和它们所支持的类在java.lang.annotation包中可以找到。(@Target,@Retention,@Documented,@Inherited)
- @Target:用于描述注解的使用范围(即:被描述的注解可以用在什么地方)
- @Retention:表示需要什么级别保存该注释信息,用于描述注解的生命周期 (SOURCE<CLASS<RUNTIME)
- @Documented:说明该注解将被包含在javadoc中
- @Inherited:说明子类可以继承父类中的该注解
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| public class Test02 { @MyAnnotation public void test(){} }
@Target(value = {ElementType.METHOD,ElementType.TYPE})
@Retention(value = RetentionPolicy.RUNTIME)
@Documented
@Inherited @interface MyAnnotation{}
|
1.4、自定义注解
- 使用@interface自定义注解时,自动继承了java.lang.annotation.Annotation接口
- 分析:
- @interface用来声明一个注解,格式:public @interface 注解名
- 其中每一个方法实际上是声明了一个配置参数
- 方法的名称就是参数的名称
- 返回值类型就是参数的类型(返回值只能是基本类型,Class,String,enum –>枚举)
- 可以通过default来声明参数的默认值
- 如果只有一个成员变量,一般参数名为value
- 注解元素必须要有值,我们定义注解元素时,经常使用空字符串,0作为默认值
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| public class Test03 { @MyAnnotation2(name = "devin",schools = {"农业大学,农大"}) public void test(){}
@MyAnnotation3("devin") public void test2(){} }
@Target({ElementType.TYPE,ElementType.METHOD}) @Retention(RetentionPolicy.RUNTIME) @interface MyAnnotation2{ String name() default ""; int age() default 0; int id() default -1;
String[] schools(); }
@Target({ElementType.TYPE,ElementType.METHOD}) @Retention(RetentionPolicy.RUNTIME) @interface MyAnnotation3{ String value(); }
|
二、反射
2.1、反射概述
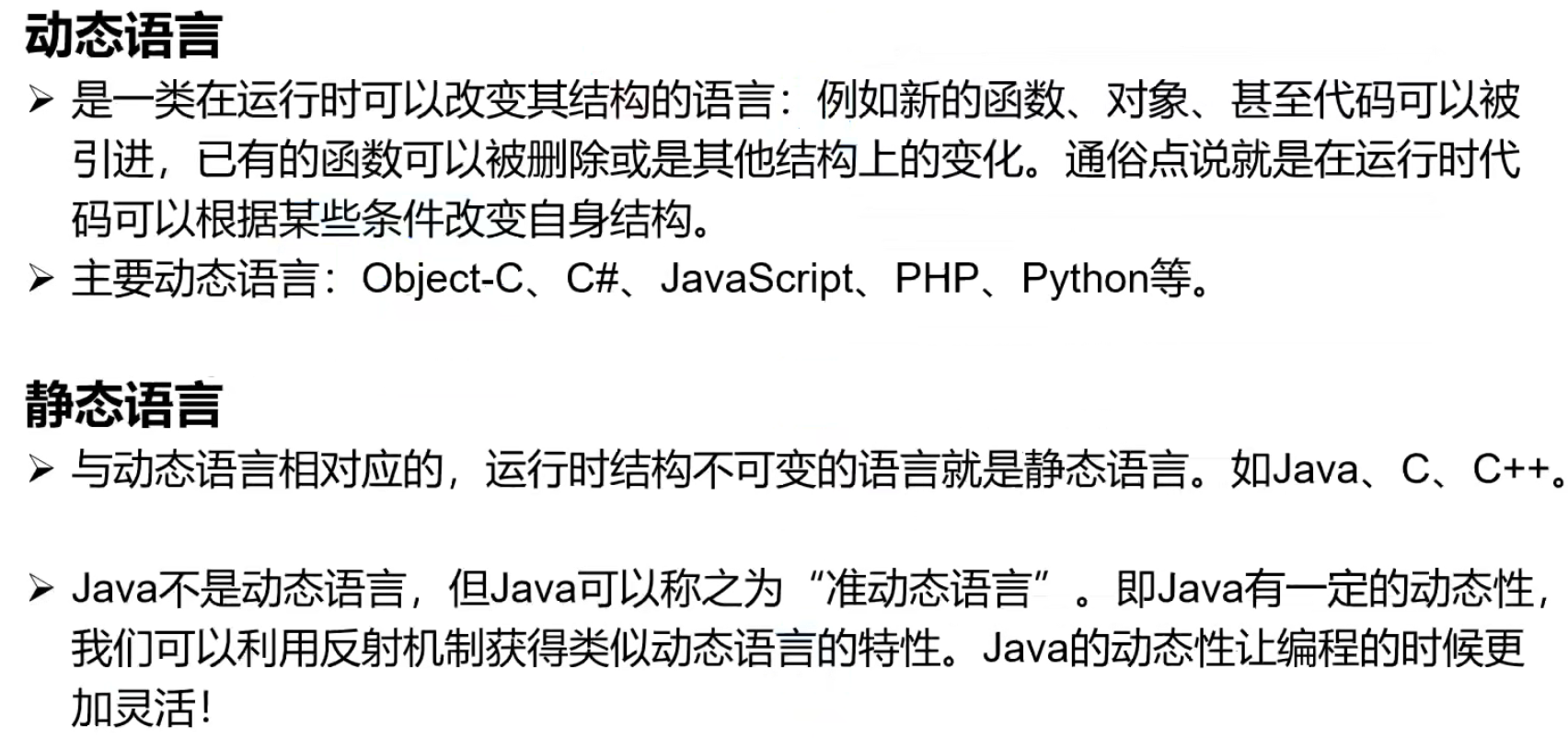
Reflection(反射)是Java被视为动态语言的关键,反射机制允许程序在执行期间借助于Reflection API取得任何类的内部信息,并能直接操作任意对象的内部属性及方法。
1
| Class c = Class.forName("java.lang.String");
|
加载完类之后,在堆内存的方法区中就产生了一个Class类型的对象(一个类只有一个Class对象),这个对象就包含了完整的类的结构信息。我们可以通过这个对象看到类的结构。这个对象就像一面镜子,透过这个镜子看到类的结构,所以,我们形象的称之为:反射!

2.2、获取反射对象
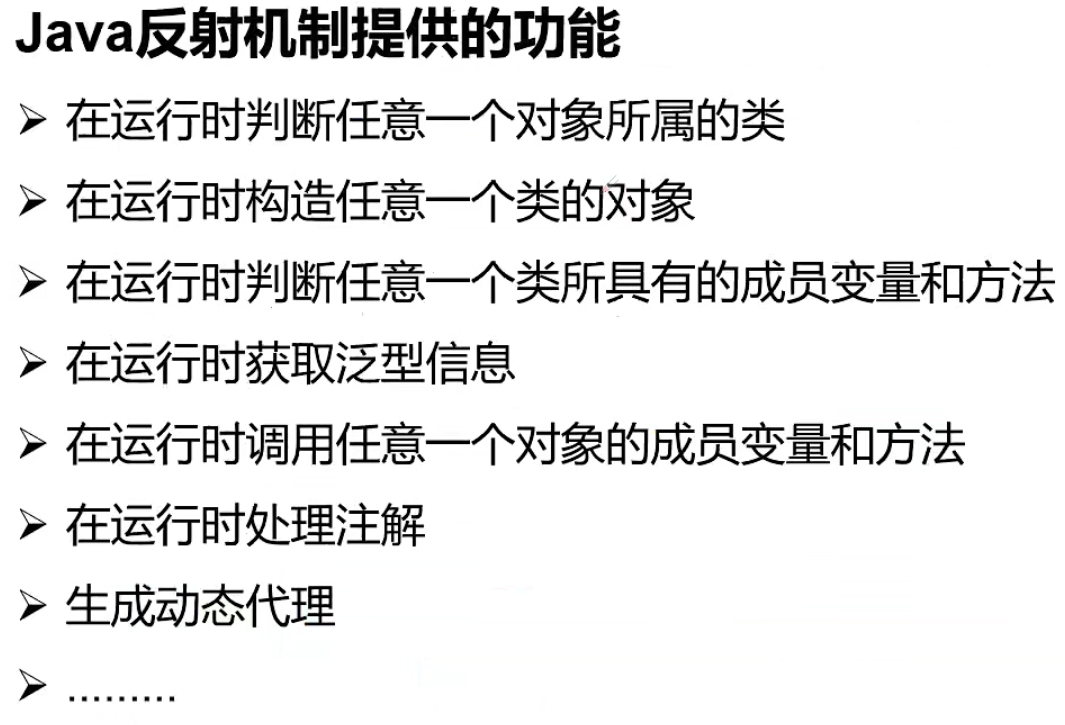
反射的优点和缺点
- 优点:可以实现动态创建对象和编译,体现出很大的灵活性
- 缺点:对性能有影响。使用反射基本上是一种解释操作,可以告诉JVM,我们希望做什么并且满足我们的要求,这类操作总是慢于直接执行相同的操作
反射相关的主要API
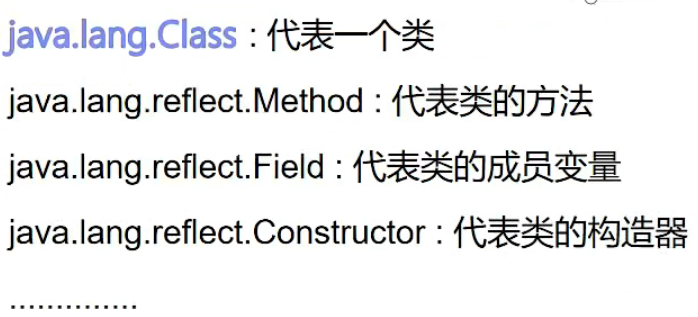
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65
| public class Test02 extends Object{ public static void main(String[] args) throws ClassNotFoundException { Class c1 = Class.forName("注解与反射.reflection.User"); System.out.println(c1); Class c2 = Class.forName("注解与反射.reflection.User"); Class c3 = Class.forName("注解与反射.reflection.User"); Class c4 = Class.forName("注解与反射.reflection.User");
System.out.println(c2.hashCode()); System.out.println(c3.hashCode()); System.out.println(c4.hashCode()); } }
class User{ private String name; private int id; private int age;
public User() { }
public User(String name, int id, int age) { this.name = name; this.id = id; this.age = age; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getId() { return id; }
public void setId(int id) { this.id = id; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
@Override public String toString() { return "User{" + "name='" + name + '\'' + ", id=" + id + ", age=" + age + '}'; } }
|
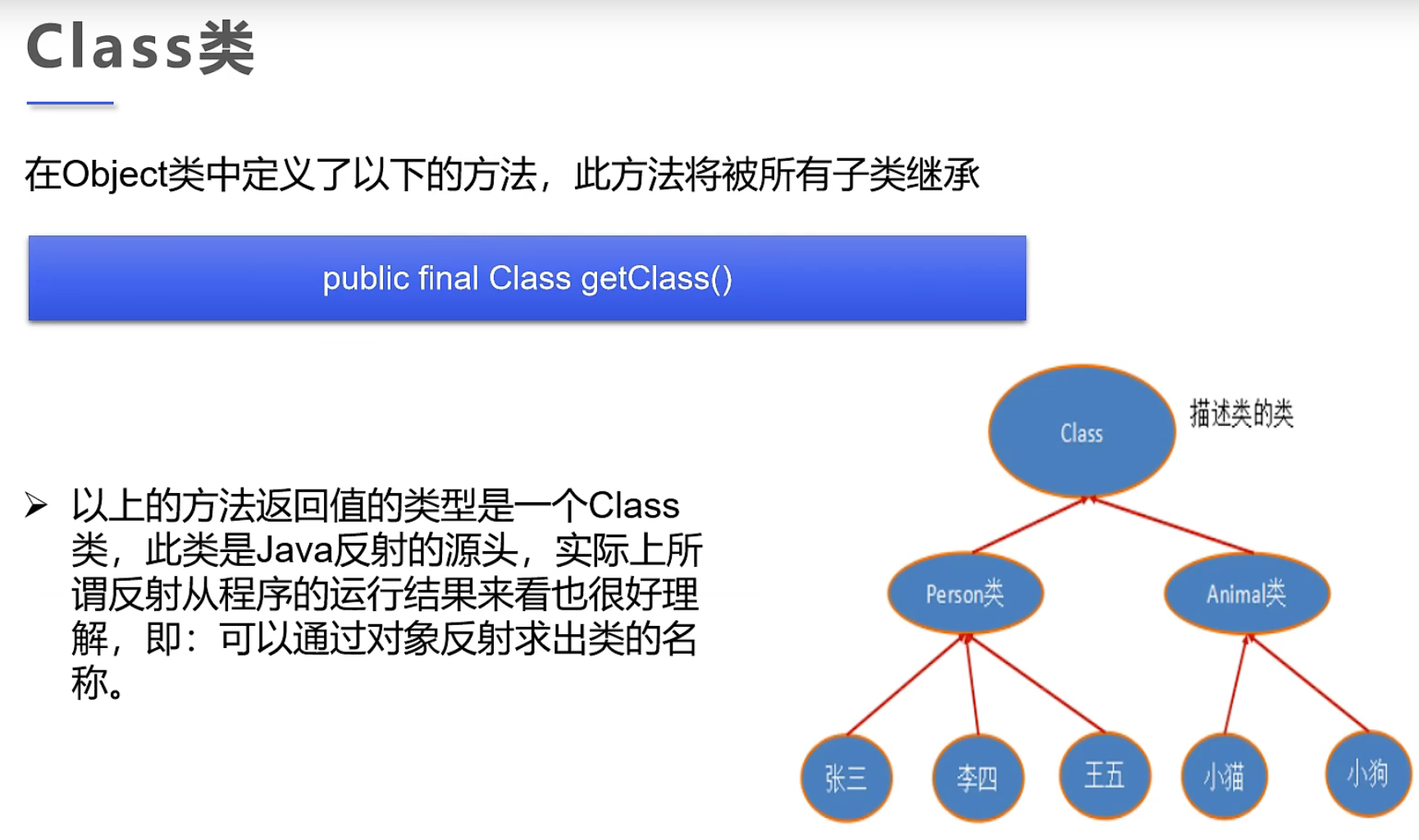
2.3、得到Class类的几种方式
Class类
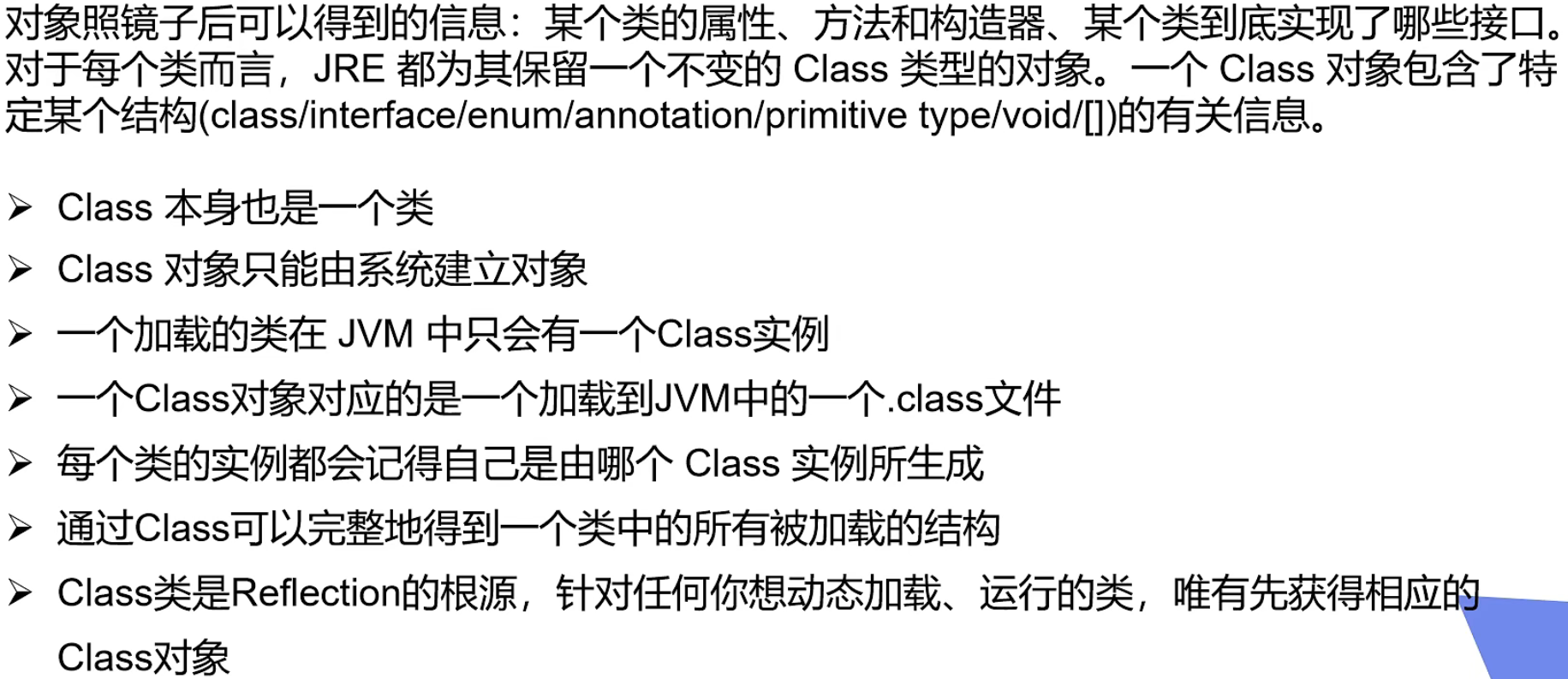
Class对象常用方法
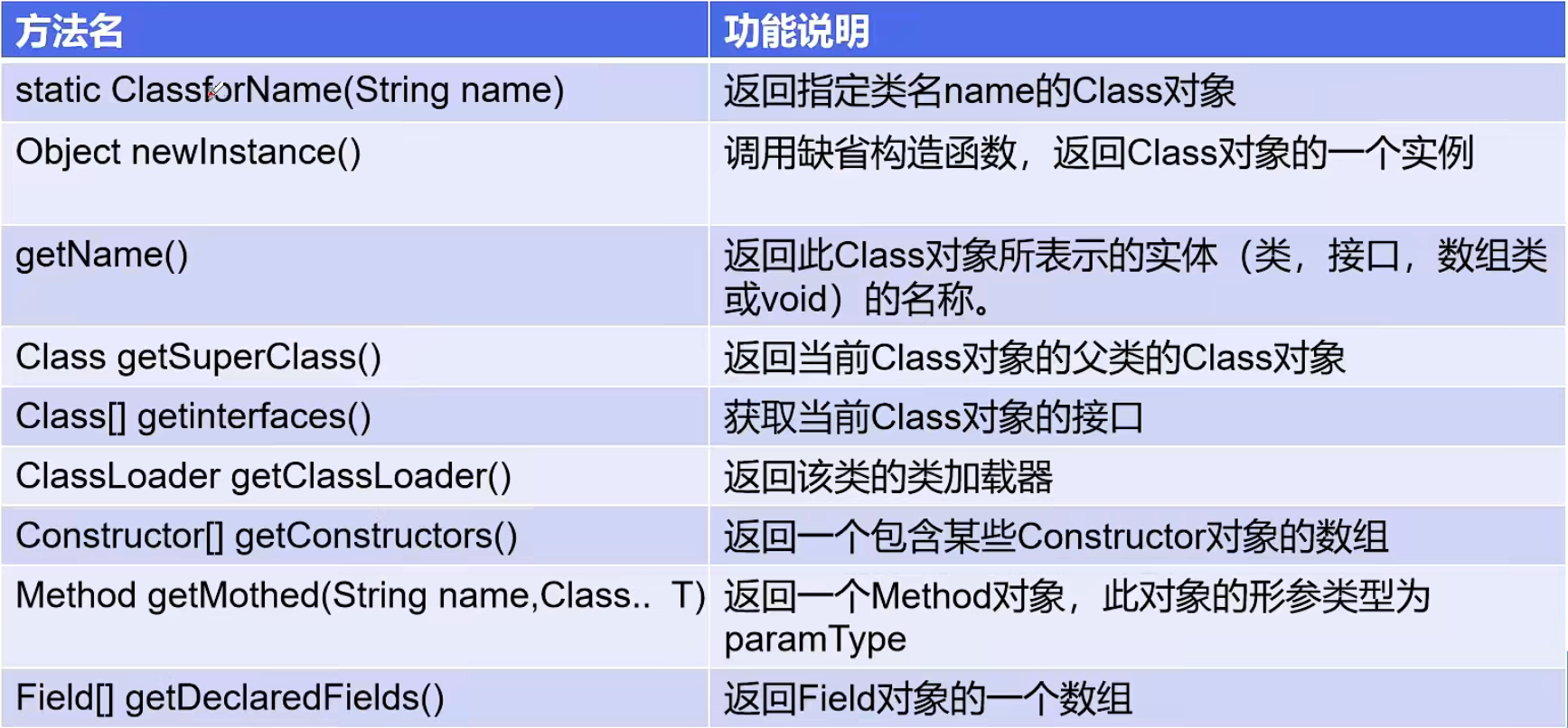
获取Class类的实例
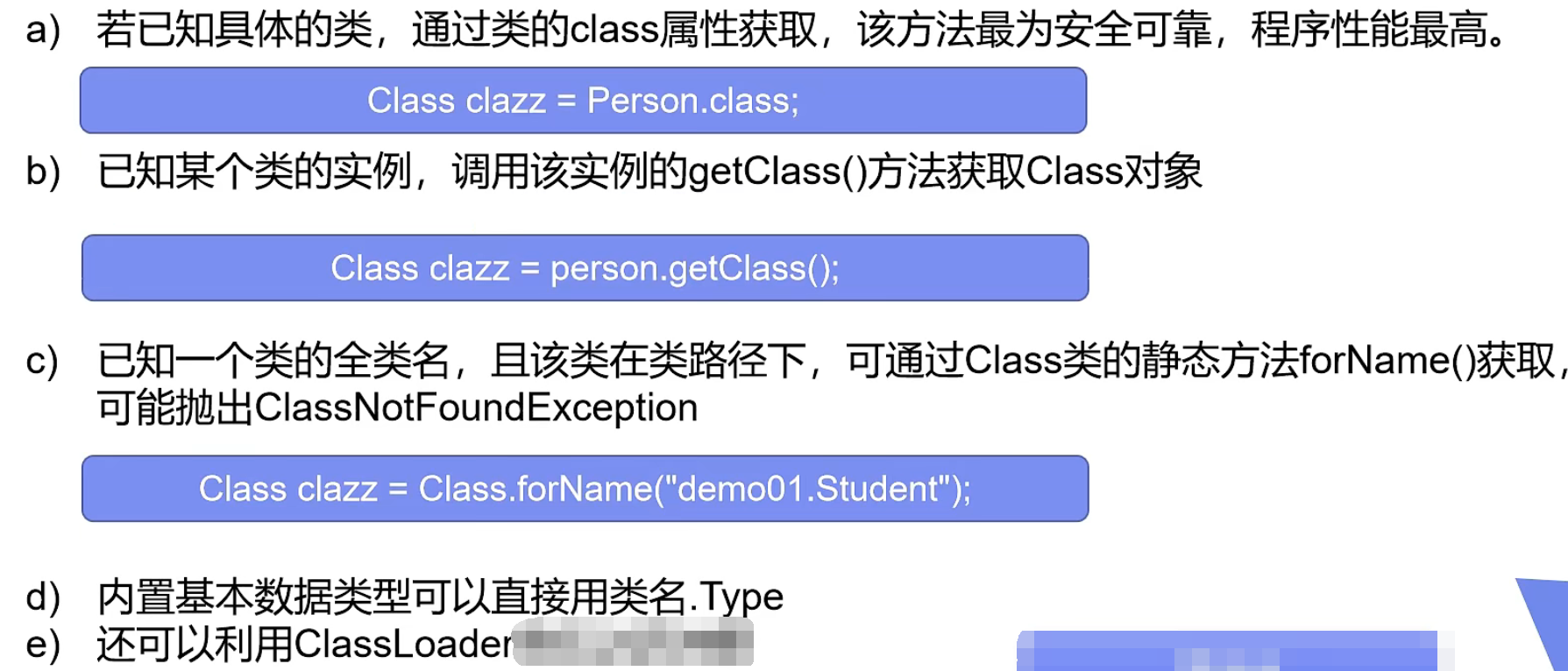
2.4、所有类型的Class对象
那些类型可以有Class对象
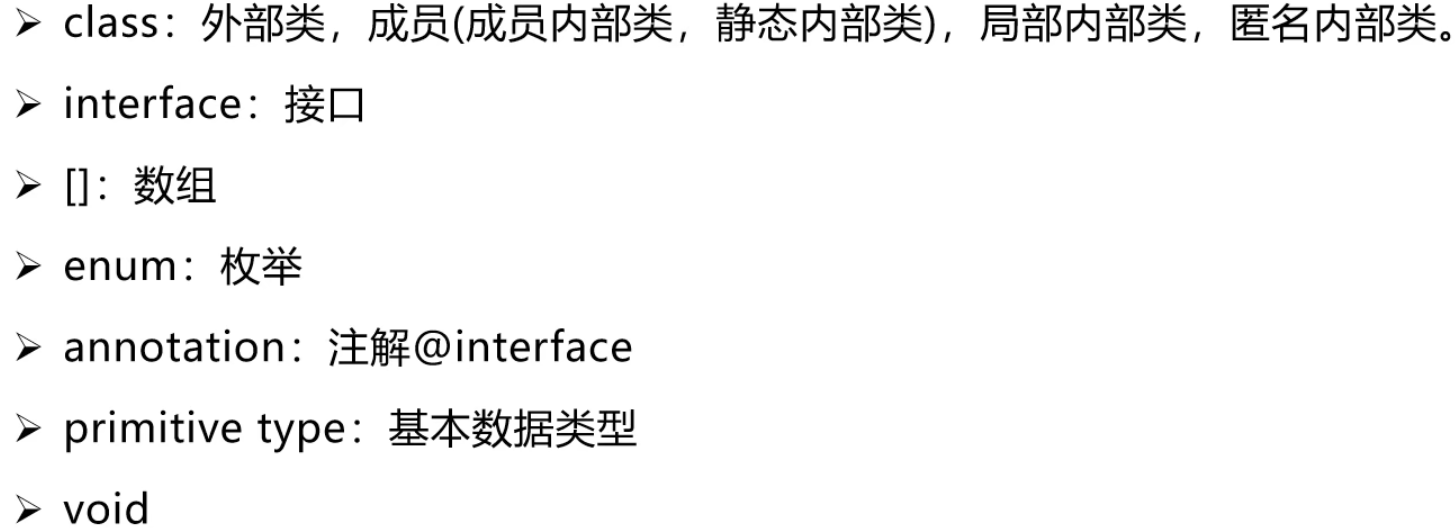
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| public class Test04 { public static void main(String[] args) { Class c1 = Object.class; Class c2 = Comparable.class; Class c3 = String[].class; Class c4 = int[][].class; Class c5 = Override.class; Class c6 = ElementType.class; Class c7 = Integer.class; Class c8 = void.class; Class c9 = Class.class;
System.out.println(c1); System.out.println(c2); System.out.println(c3); System.out.println(c4); System.out.println(c5); System.out.println(c6); System.out.println(c7); System.out.println(c8); System.out.println(c9);
int[] a = new int[10]; int[] b = new int[100]; System.out.println(a.getClass().hashCode()); System.out.println(b.getClass().hashCode()); } }
|
2.5、类加载内存分析
!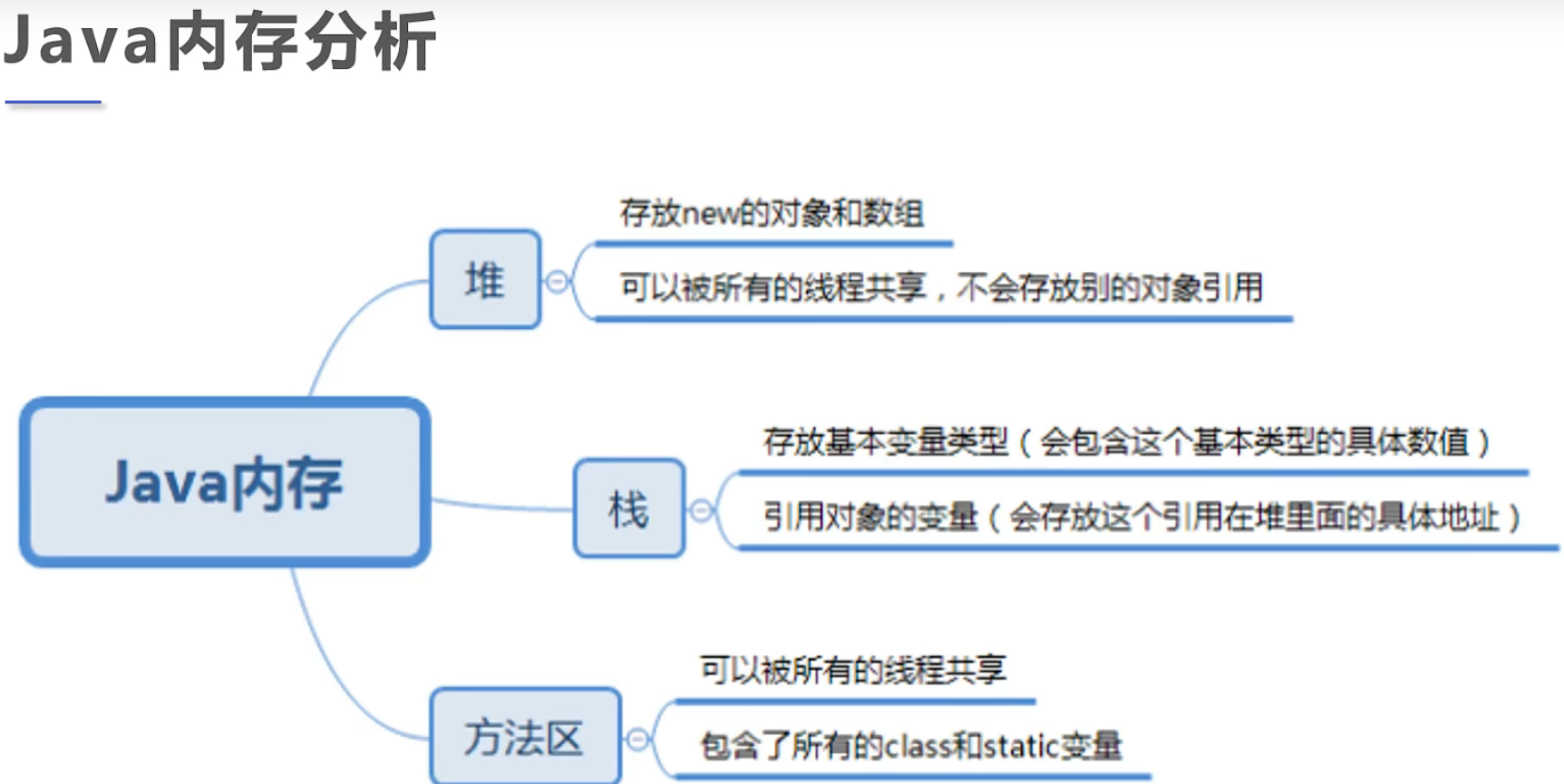
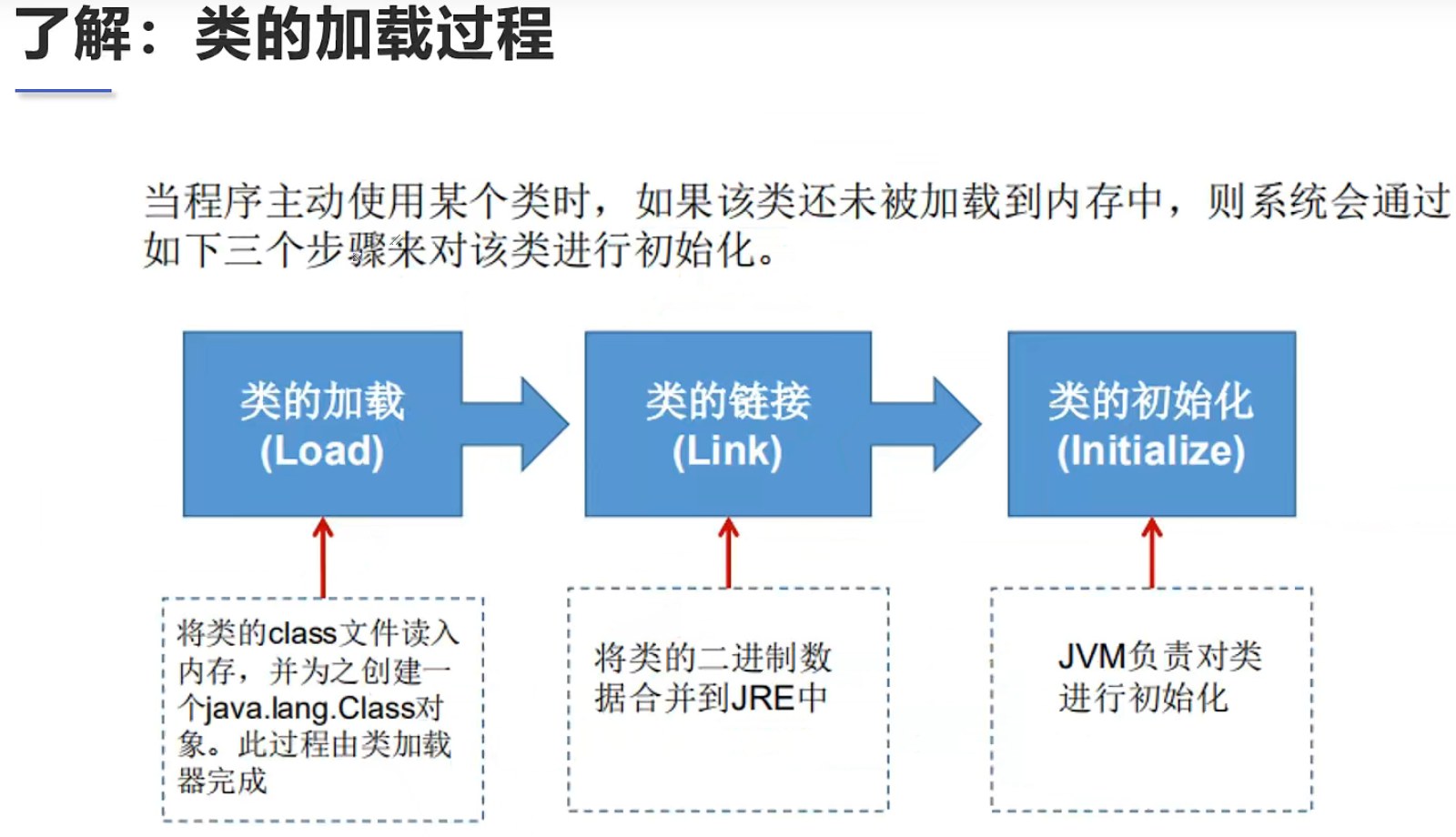
类的加载与ClassLoader的理解
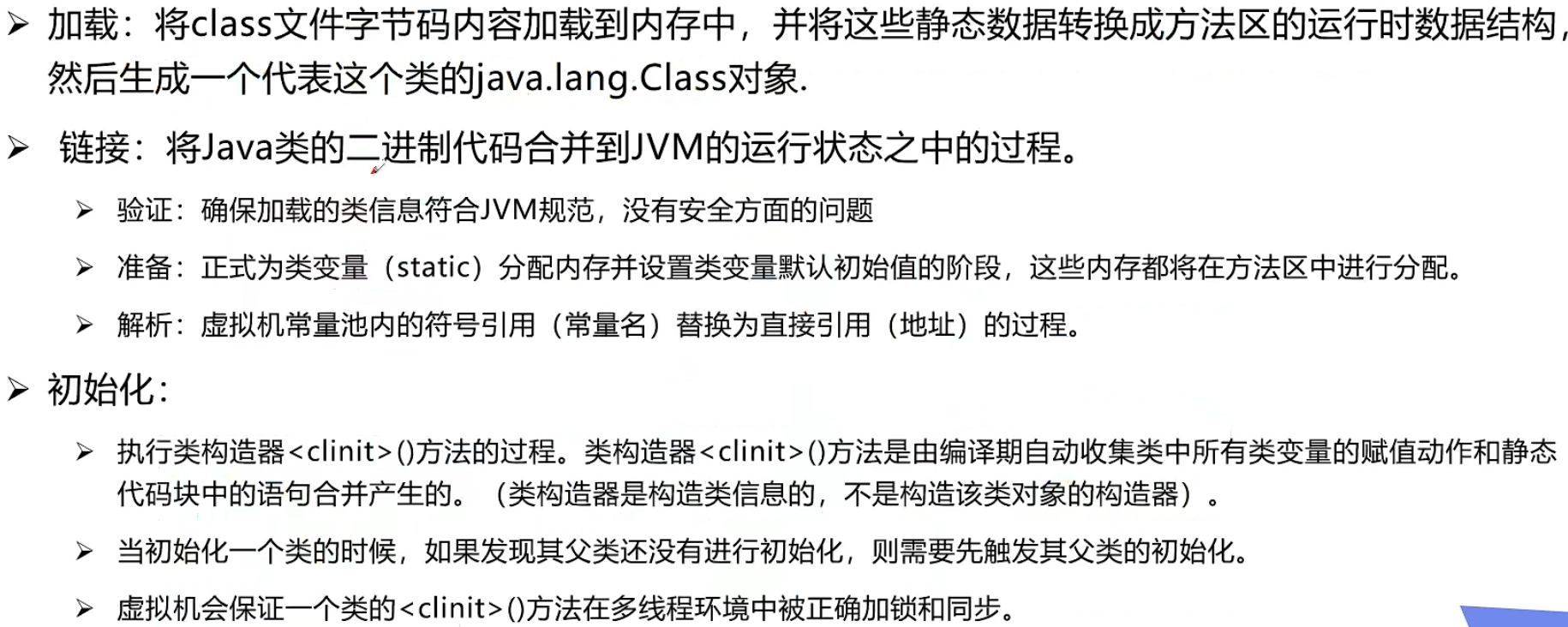
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| public class Test05 { public static void main(String[] args) { A a = new A(); System.out.println(A.m);
} }
class A{ static { System.out.println("A类静态代码块初始化"); m = 300; } static int m = 100; public A() { System.out.println("A类无参构造初始化"); } }
|
2.6、分析类的初始化
什么时候会发生类的初始化?
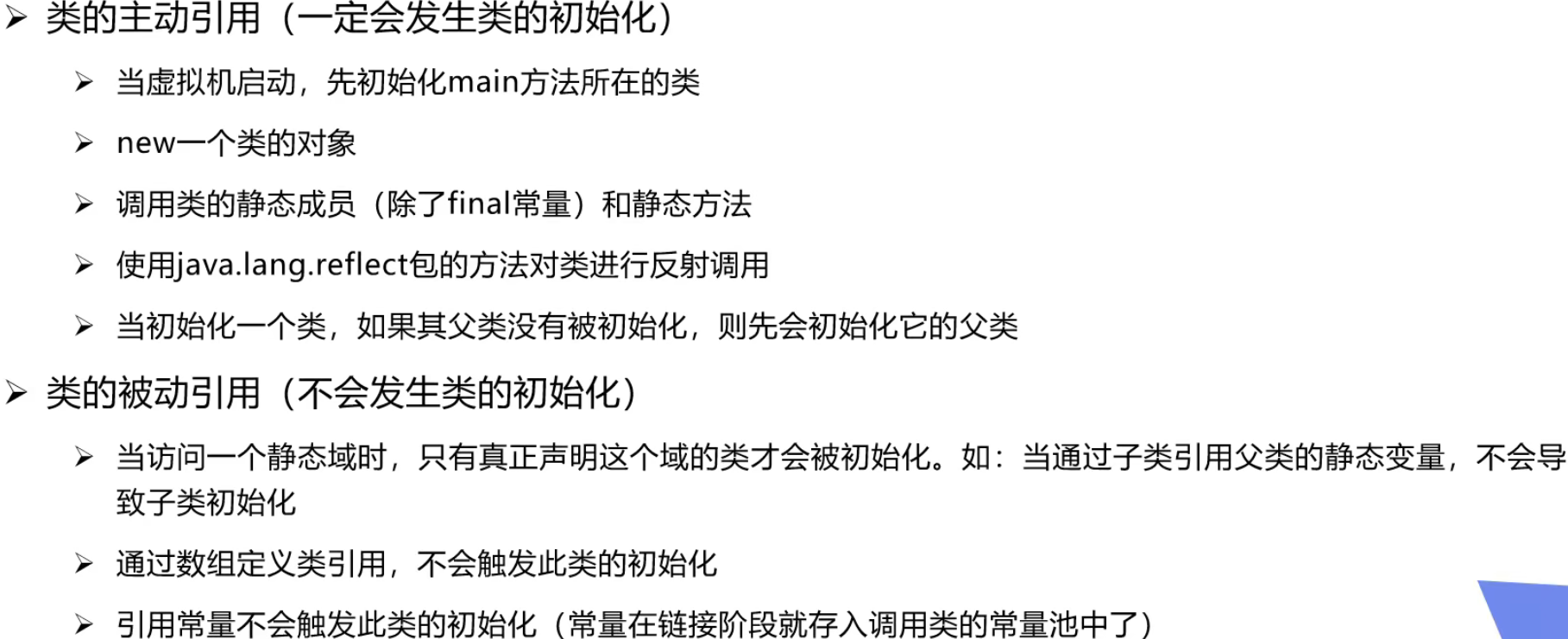
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| public class Test06 { static { System.out.println("Main被加载"); }
public static void main(String[] args) throws ClassNotFoundException {
System.out.println(Son.M);
} } class Father{ static int b = 2; static { System.out.println("父类被加载"); } }
class Son extends Father{ static { System.out.println("子类被加载"); m = 300; } static int m = 100; static final int M = 1; }
|
2.7、类加载器
类加载器的作用
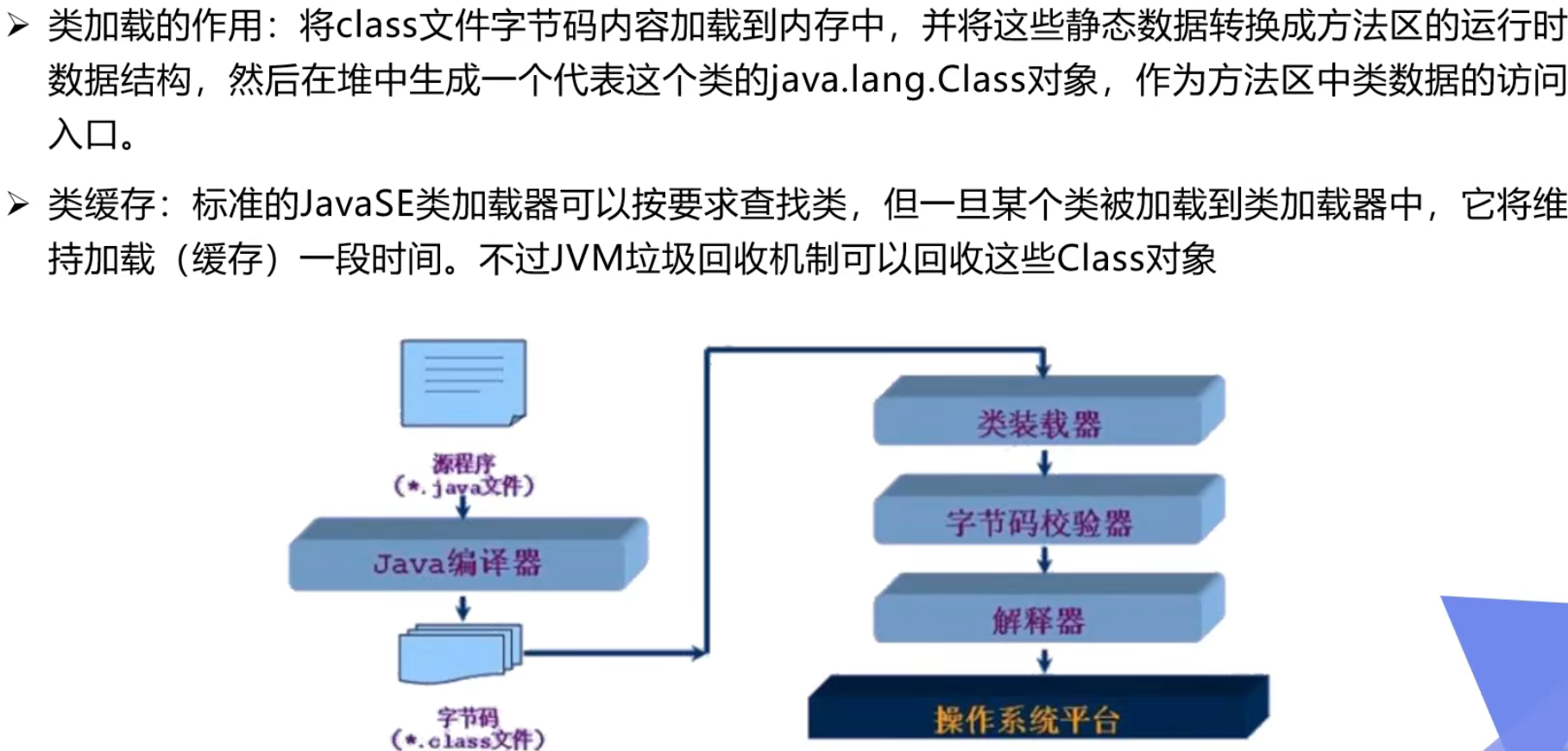
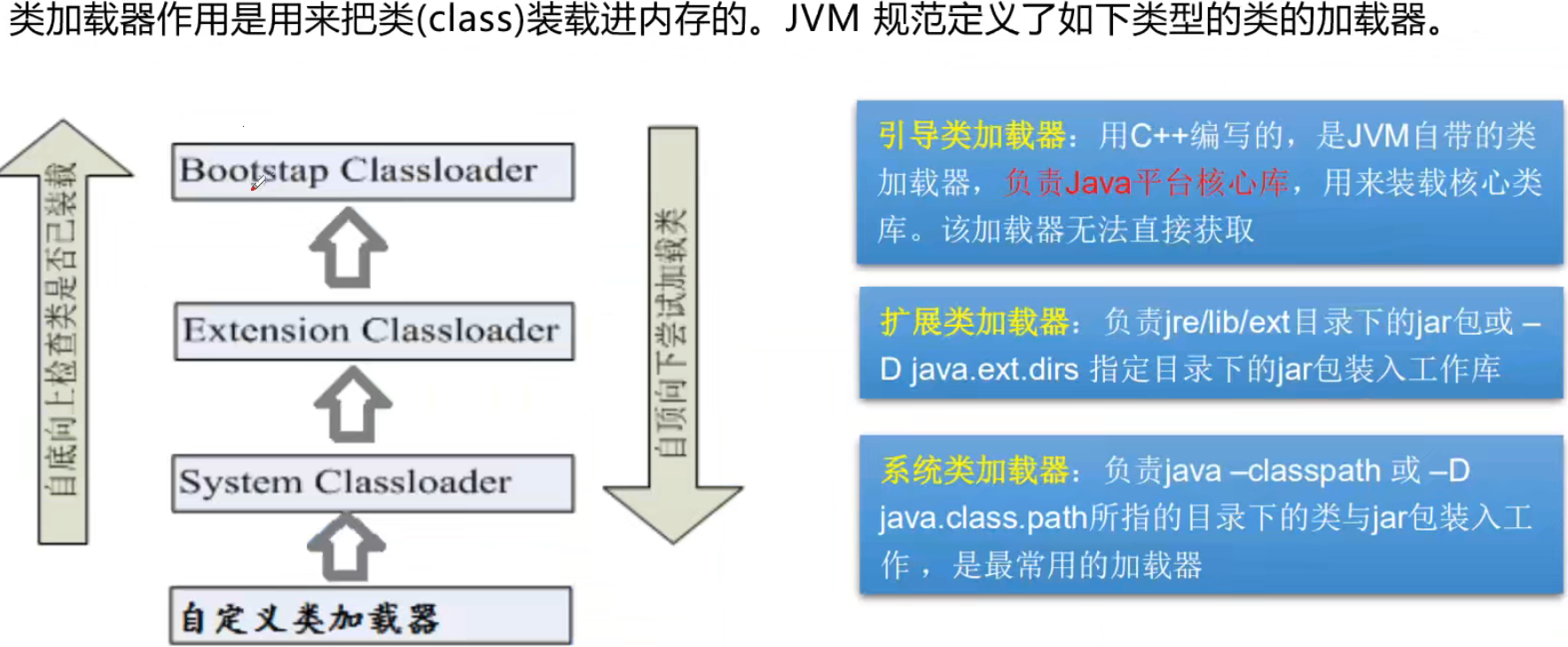
2.8、获取类的运行时结构
获取运行时类的完整结构
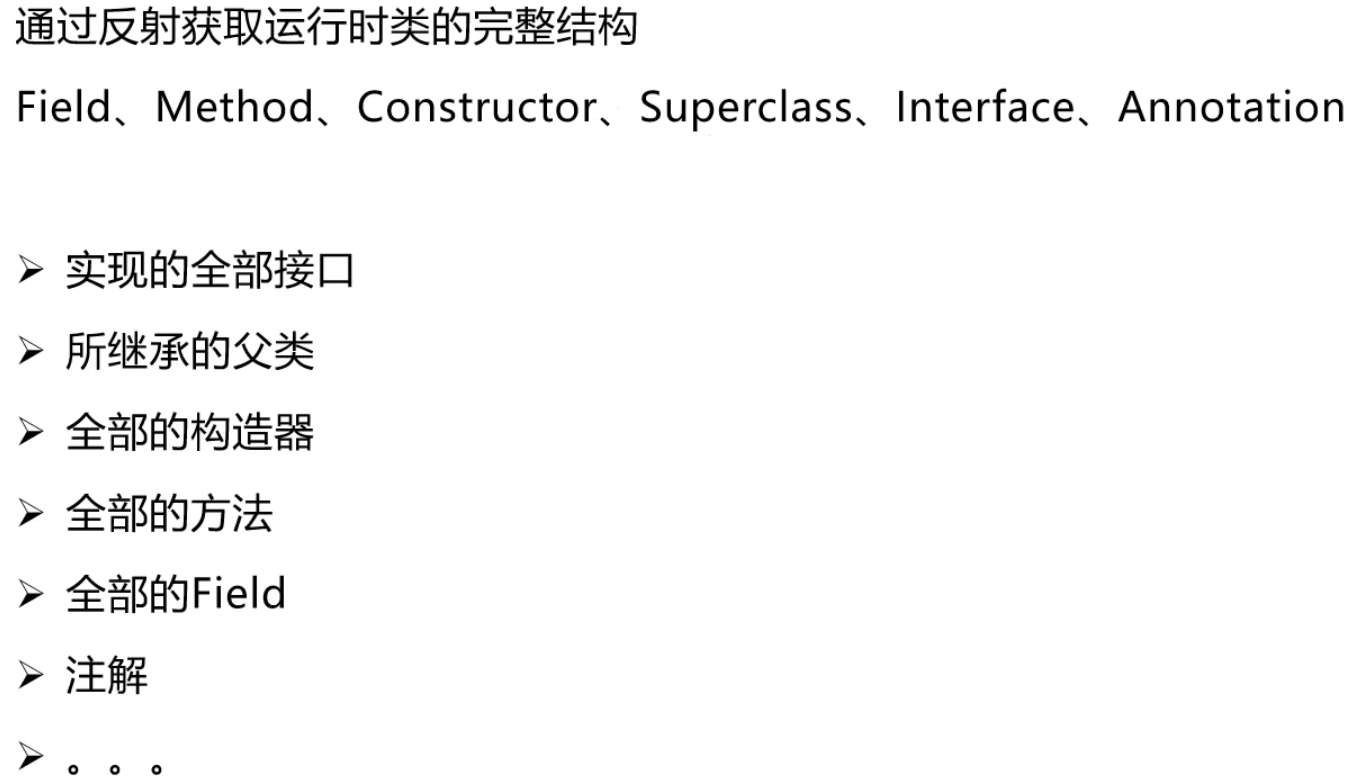
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61
| public class Test08 { public static void main(String[] args) throws ClassNotFoundException, NoSuchFieldException, NoSuchMethodException { Class c1 = Class.forName("注解与反射.reflection.User");
System.out.println(c1.getName()); System.out.println(c1.getSimpleName());
System.out.println("============================"); Field[] fields = c1.getFields();
fields = c1.getDeclaredFields(); for (Field field : fields) { System.out.println(field); }
Field name = c1.getDeclaredField("name"); System.out.println(name);
System.out.println("============================"); Method[] methods = c1.getMethods(); for (Method method : methods) { System.out.println("正常的:"+method); } methods = c1.getDeclaredMethods(); for (Method method : methods) { System.out.println("getDeclaredMethods:"+method); }
System.out.println("============================"); Method getName = c1.getMethod("getName",null); Method setName = c1.getMethod("setName",String.class); System.out.println(getName); System.out.println(setName);
System.out.println("============================"); Constructor[] constructors = c1.getConstructors(); for (Constructor constructor : constructors) { System.out.println(constructor); } constructors = c1.getDeclaredConstructors(); for (Constructor constructor : constructors) { System.out.println("#"+constructor); }
System.out.println("============================"); Constructor declaredConstructor = c1.getDeclaredConstructor(String.class, int.class,int.class); System.out.println("指定的:"+declaredConstructor); } }
|
2.9、动态创建对象执行方法
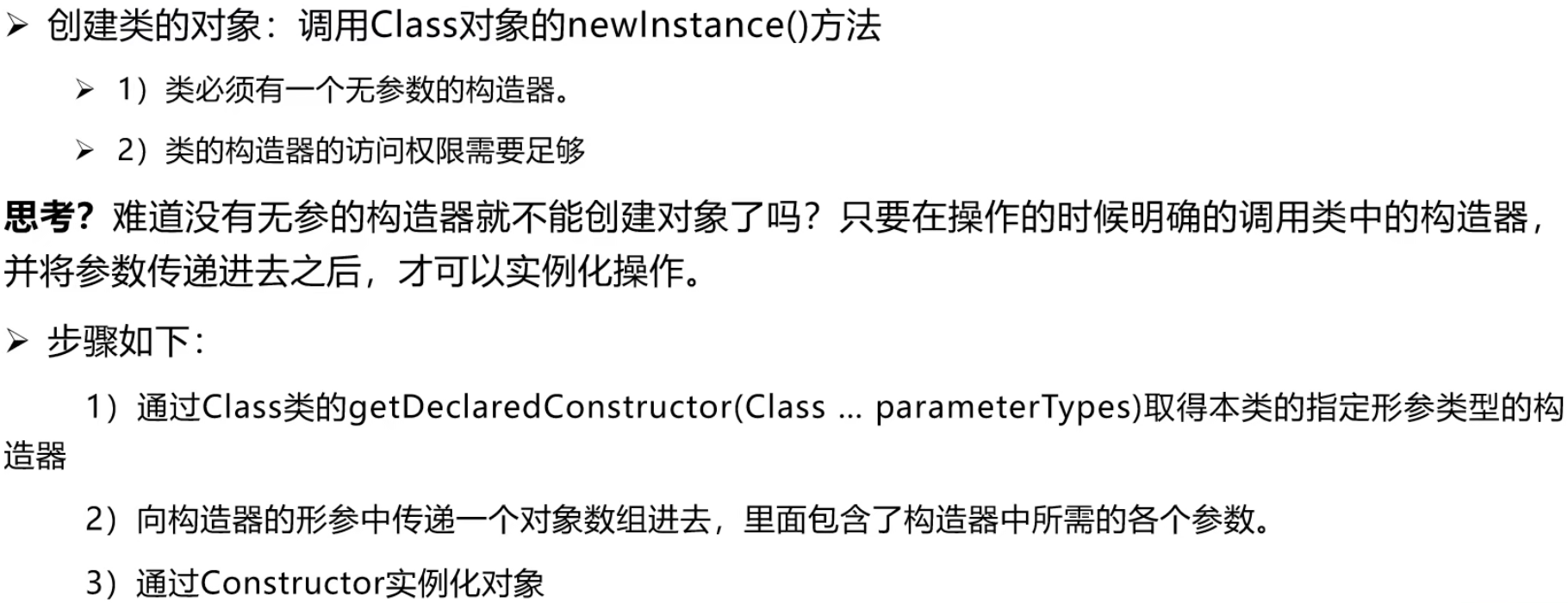
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| public class Test09 { public static void main(String[] args) throws ClassNotFoundException, IllegalAccessException, InstantiationException, NoSuchMethodException, InvocationTargetException, NoSuchFieldException { Class c1 = Class.forName("注解与反射.reflection.User");
User user3 = (User) c1.newInstance(); Method setName = c1.getDeclaredMethod("setName", String.class); setName.invoke(user3,"Devin"); System.out.println(user3.getName());
System.out.println("=========================="); User user4 = (User) c1.newInstance(); Field name = c1.getDeclaredField("name"); name.setAccessible(true); name.set(user4,"Devin2"); System.out.println(user4.getName()); } }
|
2.10、性能对比分析
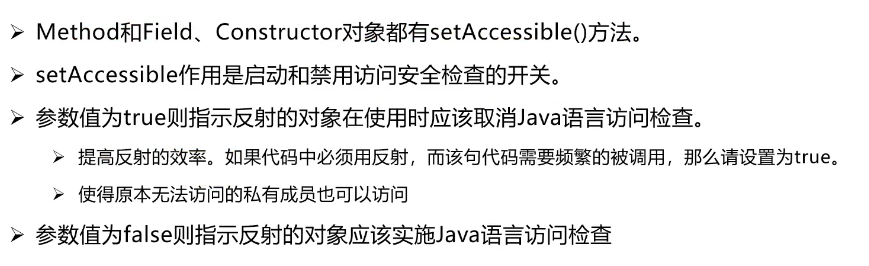
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| public class Test10 { public static void test01(){ User user = new User();
long startTime = System.currentTimeMillis(); for (int i = 0; i < 1000000000; i++) { user.getName(); } long endTime = System.currentTimeMillis(); System.out.println("普通方法执行10亿次:"+(endTime-startTime)+"ms"); }
public static void test02() throws NoSuchMethodException, InvocationTargetException, IllegalAccessException { User user1 = new User(); Class c1 = user1.getClass(); Method getName = c1.getDeclaredMethod("getName", null); long startTime = System.currentTimeMillis(); for (int i = 0; i < 1000000000; i++) { getName.invoke(user1,null); } long endTime = System.currentTimeMillis(); System.out.println("反射方式执行10亿次:"+(endTime-startTime)+"ms"); }
public static void test03() throws NoSuchMethodException, InvocationTargetException, IllegalAccessException { User user3 = new User(); Class c1 = user3.getClass(); Method getName = c1.getDeclaredMethod("getName", null); getName.setAccessible(true); long startTime = System.currentTimeMillis(); for (int i = 0; i < 1000000000; i++) { getName.invoke(user3,null); } long endTime = System.currentTimeMillis(); System.out.println("反射方式调用,关闭检测执行10亿次:"+(endTime-startTime)+"ms"); }
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException, InvocationTargetException { test01(); test02(); test03(); } }
|
2.11、获取泛型信息
反射操作泛型
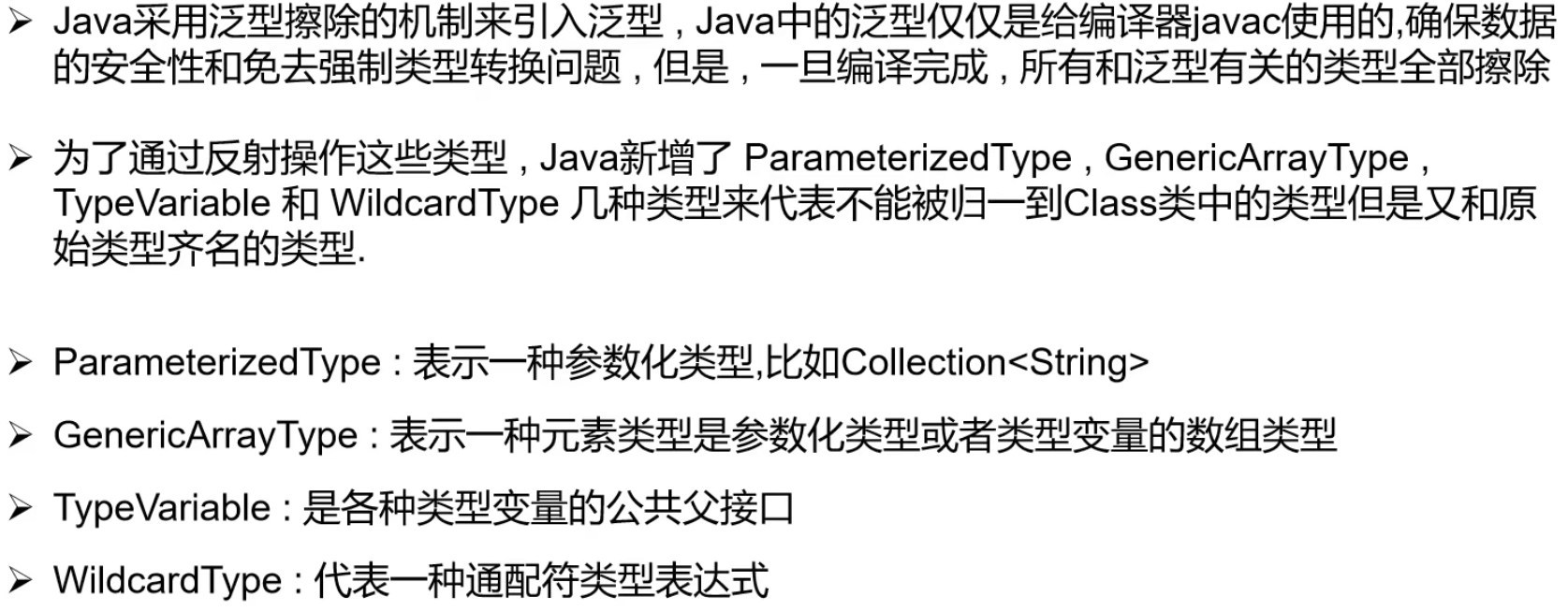
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| public class Test11 { public Test11() throws NoSuchMethodException { }
public void test01(Map<String,User> map, List<User> list){ System.out.println("test01"); }
public Map<String,User> test02(){ System.out.println("test02"); return null; }
public static void main(String[] args) throws NoSuchMethodException { Method method = Test11.class.getMethod("test01", Map.class, List.class);
Type[] genericParameterTypes = method.getGenericParameterTypes(); for (Type genericParameterType : genericParameterTypes) { System.out.println("#"+genericParameterType); if (genericParameterType instanceof ParameterizedType){ Type[] actualTypeArguments = ((ParameterizedType) genericParameterType).getActualTypeArguments(); for (Type actualTypeArgument : actualTypeArguments) { System.out.println(actualTypeArgument); } } }
method = Test11.class.getMethod("test02",null); Type genericReturnType = method.getGenericReturnType(); if (genericReturnType instanceof ParameterizedType){ Type[] actualTypeArguments = ((ParameterizedType) genericReturnType).getActualTypeArguments(); for (Type actualTypeArgument : actualTypeArguments) { System.out.println(actualTypeArgument); } } } }
|
2.12、获取注解信息
反射操作注解
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92
| public class Test12 { public static void main(String[] args) throws ClassNotFoundException, NoSuchFieldException { Class c1 = Class.forName("注解与反射.reflection.Student2");
Annotation[] annotations = c1.getAnnotations(); for (Annotation annotation : annotations) { System.out.println(annotation); }
TableDevin tableDevin = (TableDevin) c1.getAnnotation(TableDevin.class); System.out.println(tableDevin.value());
Field f = c1.getDeclaredField("name"); FileDevin annotation = f.getAnnotation(FileDevin.class); System.out.println(annotation.columnName()); System.out.println(annotation.type()); System.out.println(annotation.length()); }
}
@TableDevin("db_student") class Student2{ @FileDevin(columnName = "db_id",type = "int",length = 10) private int id; @FileDevin(columnName = "db_age",type = "int",length = 10) private int age; @FileDevin(columnName = "db_name",type = "Varchar",length = 3) private String name;
public Student2() { }
public Student2(int id, int age, String name) { this.id = id; this.age = age; this.name = name; }
public int getId() { return id; }
public void setId(int id) { this.id = id; }
public int getAge() { return age; }
public void setAge(int age) { this.age = age; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
@Override public String toString() { return "Student2{" + "id=" + id + ", age=" + age + ", name='" + name + '\'' + '}'; } }
@Target(ElementType.TYPE) @Retention(RetentionPolicy.RUNTIME) @interface TableDevin{ String value(); }
@Target(ElementType.FIELD) @Retention(RetentionPolicy.RUNTIME) @interface FileDevin{ String columnName(); String type(); int length(); }
|
转载请注明来源,欢迎对文章中的引用来源进行考证,欢迎指出任何有错误或不够清晰的表达。可以在下面评论区评论